The Best Way to Deep Clone an Object in JavaScript
This post shows you several ways to create a deep copy of an object in JavaScript.
If you want to clone an object deep in JavaScript, there are several ways to do it.
The modern way
With the structuredClone API there is a new possibility to clone deep objects.
const myExampleObject = {
name: 'Max',
age: 30,
hobbies: ['Sports', 'Cooking'],
address: {
street: 'Mainstreet 1',
city: 'Berlin',
},
};
const myExampleObjectClone = structuredClone(myExampleObject);
structuredClone() - Web APIs | MDN
The global structuredClone() method creates a deep clone of a given value using the structured clone algorithm.
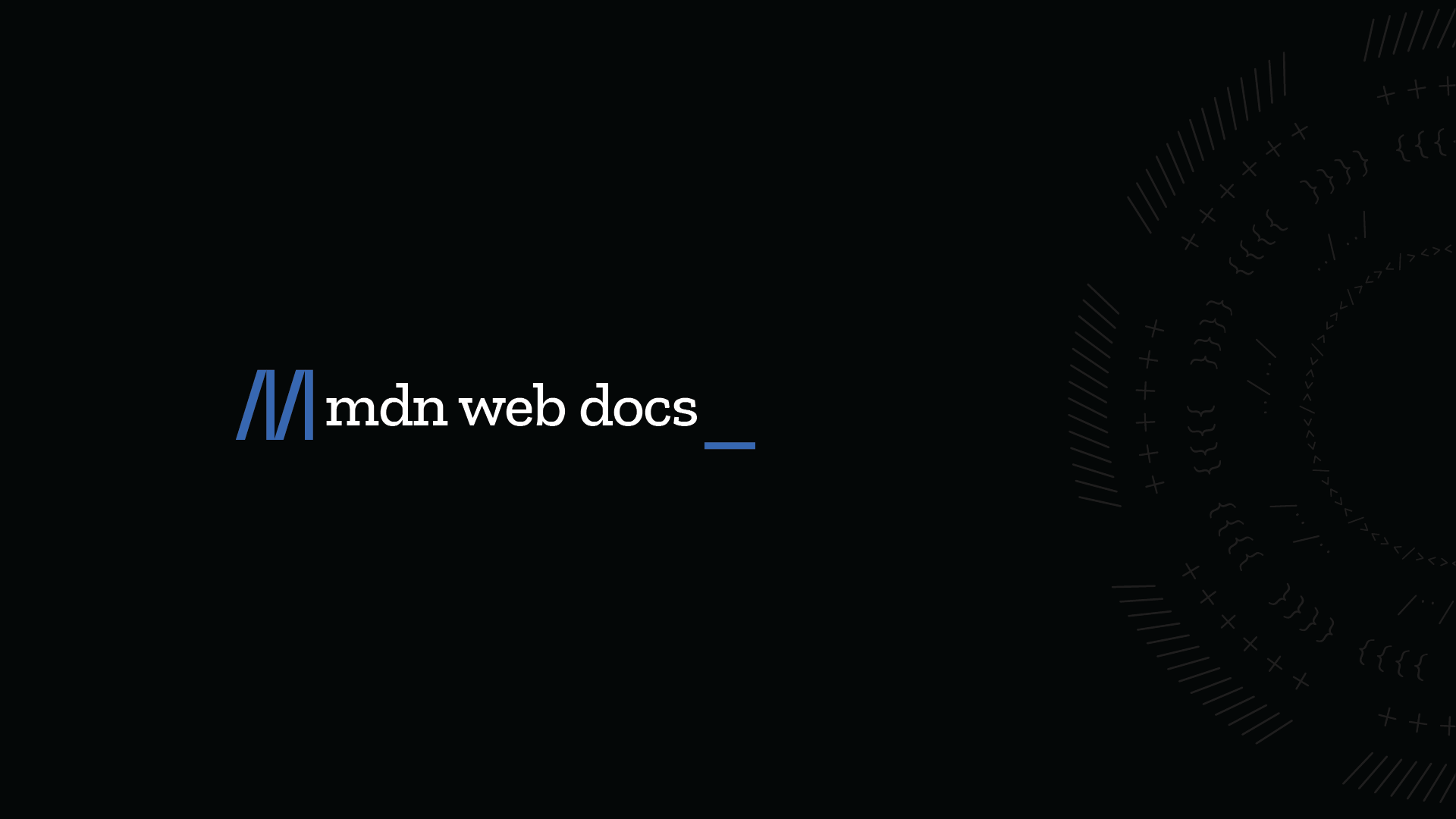
The classic way
Make a deep clone with JSON.stringify and JSON.parse.
const myExampleObject = {
name: 'Max',
age: 30,
hobbies: ['Sports', 'Cooking'],
address: {
street: 'Mainstreet 1',
city: 'Berlin',
},
};
const myExampleObjectClone = JSON.parse(JSON.stringify(myExampleObject));
The self-made way
function deepClone(obj) {
if (typeof obj !== 'object' || obj === null) {
return obj;
}
let clone = Array.isArray(obj) ? [] : {};
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
clone[key] = deepClone(obj[key]);
}
}
return clone;
}
const myExampleObject = {
name: 'Max',
age: 30,
hobbies: ['Sports', 'Cooking'],
address: {
street: 'Mainstreet 1',
city: 'Berlin',
},
};
const myExampleObjectClone = deepClone(myExampleObject);
⚠️ How a deep clone of an object doesn't work
Object.assign
Object.assign copies only the own properties of the object.
const myExampleObject = {
name: 'Max',
age: 30,
hobbies: ['Sports', 'Cooking'],
address: {
street: 'Mainstreet 1',
city: 'Berlin',
},
};
const myExampleObjectClone1 = Object.assign({}, myExampleObject);
console.log(myExampleObjectClone1 === myExampleObject); // false
console.log(myExampleObjectClone1.address === myExampleObject.address); // true
Spread syntax
The Spread syntax ... copies only the own properties of the object.
const myExampleObject = {
name: 'Max',
age: 30,
hobbies: ['Sports', 'Cooking'],
address: {
street: 'Mainstreet 1',
city: 'Berlin',
},
};
const myExampleObjectClone4 = { ...myExampleObject };
console.log(myExampleObjectClone4 === myExampleObject); // false
console.log(myExampleObjectClone4.address === myExampleObject.address); // true
Get the code for these examples with the following gist 👇
The Best Way to Deep Clone an Object in JavaScript (See my blog post: https://www.workingsoftware.dev/how-to-deep-clone-in-javascript/)
The Best Way to Deep Clone an Object in JavaScript (See my blog post: https://www.workingsoftware.dev/how-to-deep-clone-in-javascript/) - deep-clone.js
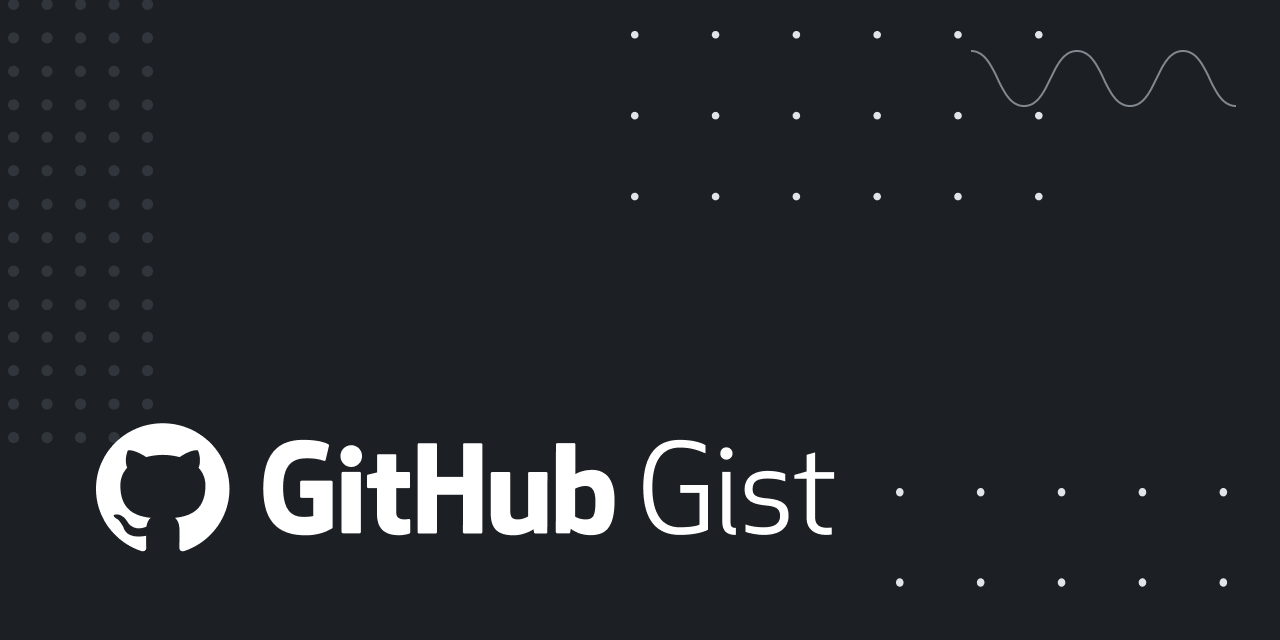
Comments ()