Understanding Enums in TypeScript and Managing Enums as Types
Unlock the power of TypeScript enums for improved code readability and strict type safety. Discover best practices for using string enums and keyof typeof for error-free development.
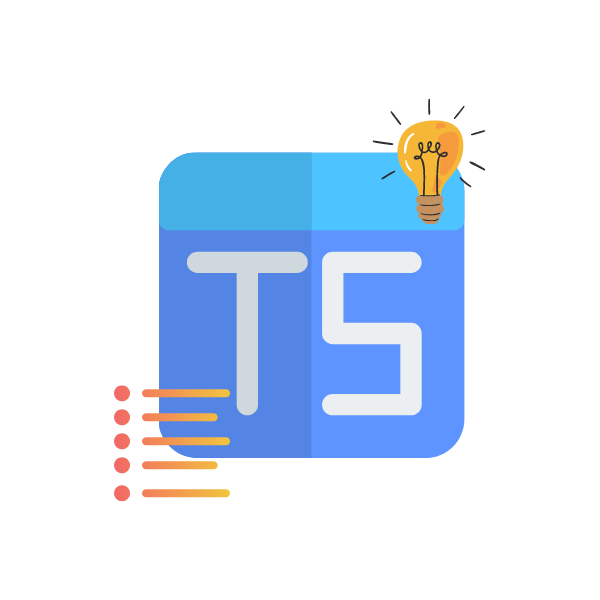
Enums, short for enumerations, are a powerful feature in TypeScript that allows developers to define a series of named constants. This can make it easier to handle a collection of related values in a more efficient and readable way.
TypeScript offers both numeric and string-based enums, giving developers flexibility in defining their data structures.
In this blog post, we'll learn how to work with enums in TypeScript. In particular, we'll focus on string-based enums and use a practical example to show how to treat them as types.
String Enums in TypeScript
String enums make it possible to assign a more meaningful value to each member, enhancing the readability of your code. Unlike numeric enums, string enums are not automatically incremented and therefore require each member to be initialized with a string value.
Here's a basic example of a string enum:
enum Direction {
Up = "Up",
Down = "Down",
Left = "Left",
Right = "Right",
}
In this example, Direction
is an enum with four members, each associated with a specific direction as a string value.
Handling Enums as Types
A powerful feature of TypeScript is the ability to use enums as types. This means that you can declare a variable or function parameter as an enum type to ensure that it can only contain one of the enum values. Using an example that is different from the original question, let's look at how to use enums as types.
Consider the following string enum declaration for different payment methods:
export const PaymentMethod = {
CreditCard: 'CreditCard',
PayPal: 'PayPal',
BankTransfer: 'BankTransfer',
} as const;
To use this enum as a type, we can leverage the keyof typeof
operator in TypeScript. This operator allows us to extract the keys of the PaymentMethod
object as a union type.
type PaymentMethodType = keyof typeof PaymentMethod;
The PaymentMethodType
type is now equivalent to a union of string literals: 'CreditCard' | 'PayPal' | 'BankTransfer'
. This means you can use PaymentMethodType
as a type annotation to ensure that variables or function parameters can only be assigned one of the three payment methods.
Example Usage
Here's how you might use the PaymentMethodType
in a function:
function processPayment(amount: number, method: PaymentMethodType) {
console.log(`Processing a ${amount} payment via ${method}`);
}
// Correct usage
processPayment(100, PaymentMethod.CreditCard); // Works fine
// Incorrect usage, TypeScript will throw an error
processPayment(100, "Check"); // Error: Argument of type '"Check"' is not assignable to parameter of type 'PaymentMethodType'.
In this example, the processPayment
function expects a payment amount and a payment method that must be one of the predefined methods in PaymentMethod
. By using PaymentMethodType
as the type for the method
parameter, TypeScript ensures type safety, allowing only the values defined in PaymentMethod
to be passed in.
Conclusion
Enums in TypeScript provide a robust way to work with a set of related constants. By using string enums and leveraging TypeScript's type system with constructs like keyof typeof
, developers can create readable and type-safe code.
The ability to treat enums as types increases the utility of TypeScript in development and ensures that your code adheres to the defined constraints, reducing the likelihood of errors.
Whether you're managing payment methods, directions, or other related values, enums and their ability to handle types are invaluable tools in the TypeScript developer's toolbox.
Comments ()